Overview
This project explores parallel computing techniques to optimize brute-force password cracking. The implementation includes two approaches: a nested loop method and a recursive algorithm, both optimized with OpenMP parallelization to enhance performance.
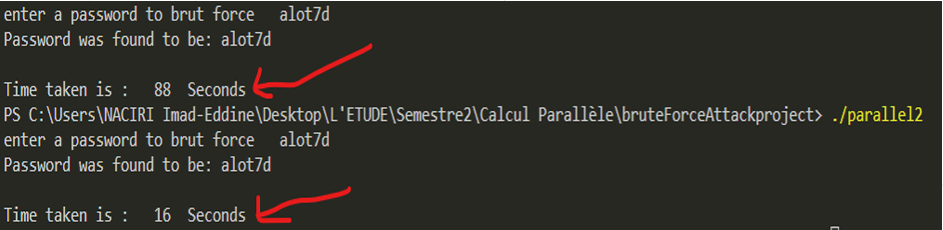
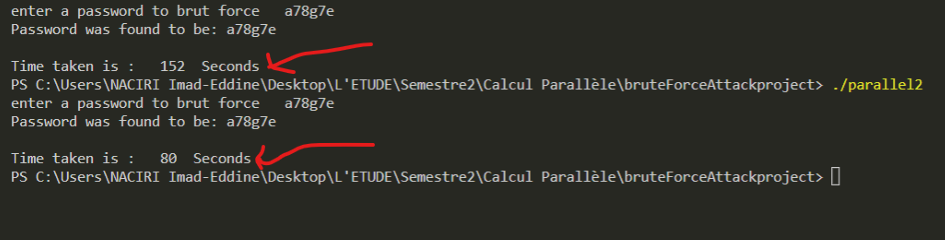
Implementation Details
-
Brute-Force Password Cracking
- Simulates a brute-force attack by generating all possible character combinations.
- Tests passwords of lengths between 2 to 6 characters.
-
Nested Loop Method (First Approach)
- Uses multiple nested loops to generate passwords sequentially.
- Parallelized within the main function for optimal performance.
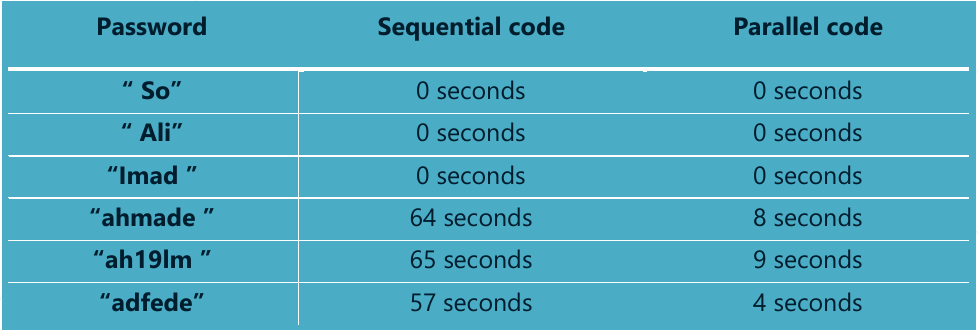
- Recursive Method (Second Approach)
- Utilizes recursion to build possible password combinations dynamically.
- Parallelized within the main function using OpenMP.
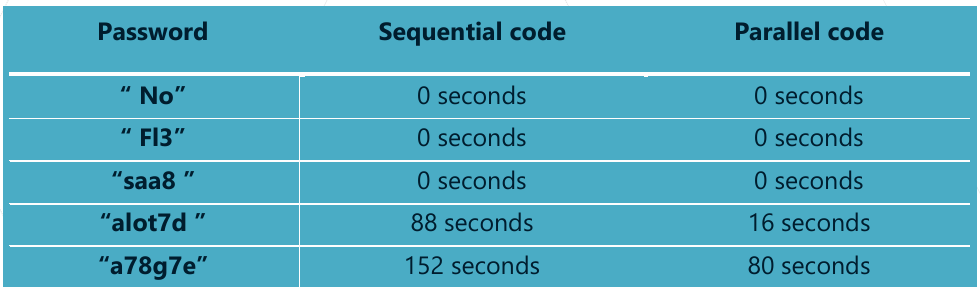
Parallelization Strategy
- Explored three parallelization methods:
- Parallelizing only within functions.
- Parallelizing only in the main function (best performance).
- Parallelizing both main and functions.
- The most efficient approach was parallelizing the main function.
Performance Comparison
Results & Findings
- The nested loop method proved to be the fastest for both sequential and parallel execution.
- Parallelization using OpenMP significantly reduced execution time, achieving up to 8x speedup.
- Recursive methods performed slower but demonstrated flexibility in cracking varying password lengths.
Future Improvements
- Extend the algorithm to support longer passwords with GPU acceleration.
- Implement optimized hash-based brute-force techniques.
- Explore hybrid approaches combining recursion and loops for better efficiency.
Contributors
- Imad-Eddine NACIRI